Designing and coding a sponsors page is part of the developer’s life (at least the lucky developer’s life, if it is about a personal site of theirs). It, however, follows different rules than those for the other pages of the site. You have to find a way to fit a lot of information and organize it clearly, so that the emphasis is put on your sponsors, and not on other elements of your design.
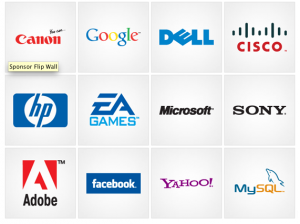
We are using PHP, CSS and jQuery with the jQuery Flip plug-in, to do just that. The resulting code can be used to showcase your sponsors, clients or portfolio projects as well.
Step 1 – XHTML
Most of the markup is generated by PHP for each of the sponsors after looping the main $sponsor array. Below you can see the code that would be generated and outputted for Google:
demo.php
01 |
< div title = "Click to flip" class = "sponsor" > |
02 |
< div class = "sponsorFlip" > |
03 |
< img alt = "More about google" src = "img/sponsors/google.png" > |
06 |
< div class = "sponsorData" > |
07 |
< div class = "sponsorDescription" > |
08 |
The company that redefined web search. |
10 |
< div class = "sponsorURL" > |
The outermost .sponsor div contains two additional div elements. The first – sponsorFlip – contains the company logo. Every click on this element causes the Flip effect to be initiated, as you will see in the jQuery part of the tutorial.
Maybe more interesting is the sponsorData div. It is hidden from view with a display:none CSS rule, but is accessible to jQuery. This way we can pass the description and the URL of the sponsoring company to the front end. After the flipping animation is complete, the contents of this div is dynamically inserted intosponsorFlip.

Sponsor Flip Wall
Step 2 – CSS
We can start laying down the styling of the wall, as without it there is no much use of the page. The code is divided in two parts. Some classes are omitted for clarity. You can see all the styles used by the demo instyles.css in the download archive.
styles.css – Part 1
02 |
/* Setting default text color, background and a font stack */ |
05 |
background-color : #fff ; |
06 |
font-family : Arial , Helvetica , sans-serif ; |
19 |
/* Giving the sponsor div a relative positioning: */ |
25 |
/* The sponsor div will be positioned absolutely with respect |
26 |
to its parent .sponsor div and fill it in entirely */ |
33 |
border : 1px solid #ddd ; |
34 |
background : url ( "img/background.jpg" ) no-repeat center center #f9f9f9 ; |
38 |
border : 1px solid #999 ; |
40 |
/* CSS3 inset shadow: */ |
41 |
-moz-box-shadow: 0 0 30px #999 inset ; |
42 |
-webkit-box-shadow: 0 0 30px #999 inset ; |
43 |
box-shadow: 0 0 30px #999 inset ; |
After styling the sponsor and sponsorFlip divs, we add a :hover state for the latter. We are using CSS3 inset box-shadow to mimic the inner shadow effect you may be familiar with from Photoshop. At the moment of writing inset shadows only work in the latest versions of Firefox, Opera and Chrome, but being primarily a visual enhancement, without it the page is still perfectly usable in all browsers.
styles.css – Part 2
02 |
/* Centering the logo image in the middle of the .sponsorFlip div */ |
07 |
margin : -70px 0 0 -70px ; |
11 |
/* Hiding the .sponsorData div */ |
17 |
padding : 50px 10px 20px 20px ; |
28 |
/* This class clears the floats */ |
As mentioned earlier, the sponsorData div is not meant for viewing, so it is hidden with display:none. Its purpose is to only store the data which is later extracted by jQuery and displayed at the end of the flipping animation.

Flipping Animation
Step 3 – PHP
You have many options in storing your sponsors data – in a MySQL database, XML document or even a plain text file. These all have their benefits and we’ve used all of them in the previous tutorials (except XML storage, note to self).
However, the sponsor data is not something that changes often. This is why a different approach is needed. For the purposes of the task at hand, we are using a multidimensional array with all the sponsor information inside it. It is easy to update and even easier to implement:
demo.php – Part 1
01 |
// Each sponsor is an element of the $sponsors array: |
21 |
// Randomizing the order of sponsors: |
The sponsors are grouped into the main $sponsors array. Each sponsor entry is organized as a separate regular array. The first element of that array is the unique key of the sponsor, which corresponds to the file name of the logo. The second element is a description of the sponsor and the last is a link to the sponsor’s website.
After defining the array, we use the in-build shuffle() PHP function to randomize the order in which the sponsors are displayed.
demo.php – Part 2
01 |
// Looping through the array: |
03 |
foreach ( $sponsors as $company ) |
06 |
<div class = "sponsor" title= "Click to flip" > |
07 |
<div class = "sponsorFlip" > |
08 |
<img src= "img/sponsors/'.$company[0].'.png" alt= "More about '.$company[0].'" /> |
11 |
<div class = "sponsorData" > |
12 |
<div class = "sponsorDescription" > |
15 |
<div class = "sponsorURL" > |
16 |
<a href= "'.$company[2].'" > '.$company[2].' </a> |
The code above can be found halfway down demo.php. It basically loops through the shuffled $sponsorsarray and outputs the markup we discussed in step one. Notice how the different elements of the array are inserted into the template.
Step 4 – jQuery
The jQuery Flip plugin requires both the jQuery library and jQuery UI. So, after including those in the page, we can move on with writing the code that will bring our sponsor wall to life.
script.js
01 |
$(document).ready( function (){ |
02 |
/* The following code is executed once the DOM is loaded */ |
04 |
$( '.sponsorFlip' ).bind( "click" , function (){ |
06 |
// $(this) point to the clicked .sponsorFlip element (caching it in elem for speed): |
10 |
// data('flipped') is a flag we set when we flip the element: |
12 |
if (elem.data( 'flipped' )) |
14 |
// If the element has already been flipped, use the revertFlip method |
15 |
// defined by the plug-in to revert to the default state automatically: |
19 |
// Unsetting the flag: |
20 |
elem.data( 'flipped' , false ) |
24 |
// Using the flip method defined by the plugin: |
30 |
// Insert the contents of the .sponsorData div (hidden |
31 |
// from view with display:none) into the clicked |
32 |
// .sponsorFlip div before the flipping animation starts: |
34 |
elem.html(elem.siblings( '.sponsorData' ).html()); |
39 |
elem.data( 'flipped' , true ); |
First we bind a function as a listener for the click event on the .sponsorFlip divs. After a click event occurs, we check whether the flipped flag is set via the jquery data() method. This flag is set individually for each sponsorFlip div and helps us determine whether the div has already been flipped. If this is so, we use the revertFlip() method which is defined by the Flip plugin. It returns the div to its previous state.
If the flag is not present, however, we initiate a flip on the element. As mentioned earlier, the .sponsorDatadiv, which is contained in every sponsor div, contains the description and the URL of the sponsor, and is hidden from view with CSS. Before the flipping starts, the plug-in executes the onBefore function we define in the configuration object that is passed as a parameter (line 29). In it we change the content of thesponsorFlip div to the one of sponsorData div, which replaces the logo image with information about the sponsor.
With this our sponsor flip wall is complete!
Conclusion
Today we used the jQuery Flip plug-in to build a sponsor wall for your site. You can use this example to bring interactivity to your site’s pages. And as the data for the wall is read from an array, you can easily modify it to work with any kind of database or storage.
What do you think? How would you modify this code?
Source
http://tutorialzine.com/2010/03/sponsor-wall-flip-jquery-css/